SingleStore Kai
On this page
Use the SingleStore Kai ("the API") to connect to a SingleStore Helios workspace from MongoDB® clients and tools.
Refer to Maintenance Release Changelog for information on features and improvements introduced since the preview release of SingleStore Kai.
Note
You can only run the MongoDB® commands in your SingleStore Helios database using the SingleStore Kai-enabled endpoint.
Disclaimer:
SingleStore is not a MongoDB® partner.
Why Use the API for MongoDB®
SingleStore Kai offers an innovative, fast, and easy API to perform exceptionally fast analytics on JSON data for your MongoDB® applications without losing transactional performance.
Key benefits to using SingleStore Kai:
-
Drive faster analytics on JSON data for your MongoDB® applications.
-
Zero code changes and zero data transformations required for supported commands and operations.
-
Best of both worlds (NoSQL + SQL), lets developers utilize both the MongoDB® API and a SQL API to power their applications.
-
Scale-out SingleStore without any additional cost and simply by defining a shard key.
-
Vector support lets users provide semantics on vectors using
dot_
,product euclidean_
, and many other functions available through the API.distance
How the API Works
The API uses a proxy service (provided by SingleStore) to run the MongoDB® commands and aggregation pipelines.
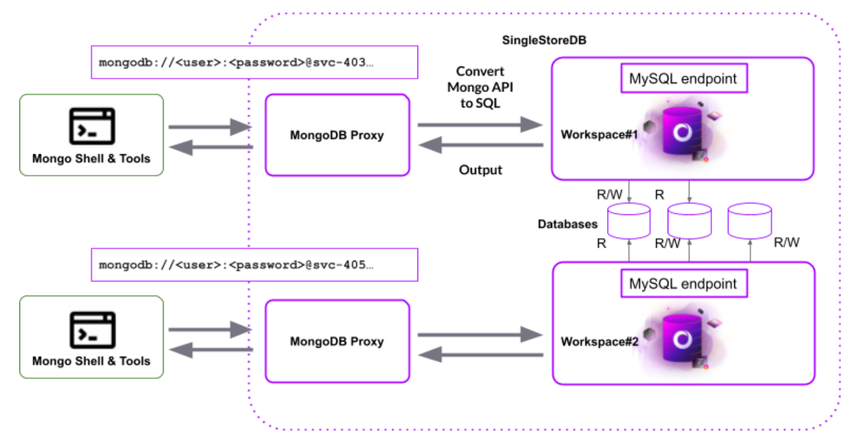
Availability
The SingleStore Kai feature is available in all the regions where SingleStore Helios can be deployed.
In this section
- Getting Started with SingleStore Kai
- Kai Data Storage Model
- Migrate from MongoDB® to SingleStore
- Replicate MongoDB® Collections to SingleStore
- SingleStore Extension Commands
- Supported MongoDB® Commands, Data Types, and Operators
- BSON Data Type Mapping
- MongoDB® to SQL Mapping
- Best Practices
- Error Handling
- Limitations
- Maintenance Release Changelog
Last modified: August 2, 2024